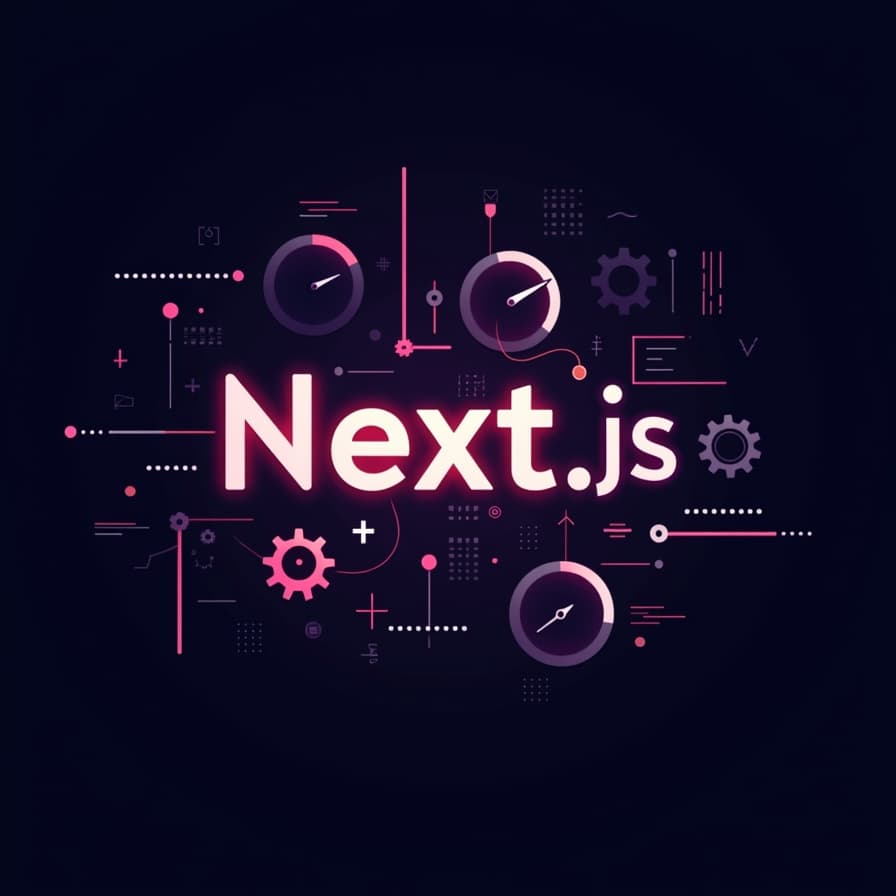
- 30 Views
How to Optimize Next.js Web Apps for Better Performance
Introduction to Next.js Performance Optimization
Next.js is a powerful React framework that allows developers to build performant web applications with ease. However, like any modern web app, it's essential to focus on optimizing performance to deliver faster load times, better user experiences, and improved SEO. In this post, we'll dive into some key strategies for improving the performance of your Next.js web apps.
Key Factors to Consider for Performance Optimization:
- Faster page load times for better user experience.
- Optimized server-side rendering (SSR) for faster content delivery.
- Efficient image and asset loading to reduce page size.
- SEO improvement through proper optimization techniques.
Enable Static Site Generation (SSG) and Incremental Static Regeneration (ISR)
Static Site Generation (SSG) and Incremental Static Regeneration (ISR) are key features of Next.js that allow you to pre-render pages at build time. By generating static HTML pages, your website can serve faster content, reducing server load and improving overall performance.
How to Use SSG and ISR in Next.js:
- Use `getStaticProps` for static generation of pages.
- Implement `getStaticPaths` with dynamic routes to generate static content.
- For dynamic content updates, use `revalidate` in ISR to update content after a specified period without rebuilding the whole site.
Optimize Images with Next.js Image Component
One of the largest contributors to slow page load times is unoptimized images. Next.js comes with an `Image` component that allows you to automatically optimize images by resizing, lazy loading, and serving them in modern formats like WebP for better performance.
How to Optimize Images in Next.js:
- Import the `next/image` module and replace `<img>` tags with the `<Image />` component.
- Next.js automatically optimizes images by serving the appropriate size based on the device's screen size.
- Use `priority` for images that appear above the fold to load them sooner.
- Leverage the `placeholder` property to display a blurred image while the actual image loads.
Leverage Code Splitting and Dynamic Imports
Code splitting ensures that only the necessary JavaScript code is loaded on each page, improving load times and reducing the size of the initial bundle. Dynamic imports allow for lazy loading of code, which helps in reducing the amount of JavaScript that needs to be parsed and executed on initial load.
How to Implement Code Splitting and Dynamic Imports in Next.js:
- Use `dynamic` from `next/dynamic` to load components lazily.
- Example: `const Component = dynamic(() => import('../components/Component'));`
- This will split the component into a separate bundle that only loads when needed.
Implement Client-Side Caching and Service Workers
Caching is a powerful technique to improve the performance of web apps by reducing the need to re-fetch data and assets on each page load. In Next.js, you can leverage service workers, the browser cache, and caching headers to enhance performance.
Caching Strategies to Optimize Performance:
- Use `Cache-Control` headers to instruct browsers to cache assets and avoid re-fetching them unnecessarily.
- Implement a service worker using the `next-offline` plugin to cache assets for offline use and improve load times.
- Leverage Next.js's automatic caching of API routes and pre-rendered pages.
Optimize Fonts for Faster Rendering
Fonts can significantly affect page load times. By using font optimization techniques, you can reduce render-blocking behavior, improve loading speeds, and enhance user experience. Next.js provides built-in features to optimize font loading and rendering.
Font Optimization in Next.js:
- Use `next/font` to import fonts and control how they are loaded.
- Implement `font-display: swap` to ensure text is visible while fonts are loading.
- Leverage Google Fonts or self-hosted fonts with proper optimizations.
Optimize API Routes and Server-Side Performance
API routes and server-side rendering (SSR) can impact performance if not properly optimized. It's essential to ensure that API routes are efficient, and server-side pages are pre-rendered correctly to minimize delays.
Tips for Optimizing API Routes and SSR in Next.js:
- Use SSR sparingly and only when necessary to avoid unnecessary server load.
- Optimize API routes by reducing complexity, leveraging caching, and handling errors gracefully.
- Consider using `getServerSideProps` for pages that require real-time data while optimizing for performance.
Conclusion
Optimizing the performance of your Next.js web apps is crucial for providing a fast and seamless user experience. By leveraging built-in features like Static Site Generation, image optimization, code splitting, and caching, you can drastically improve performance. Always monitor your web app's performance and make adjustments as needed to ensure the best possible experience for your users.