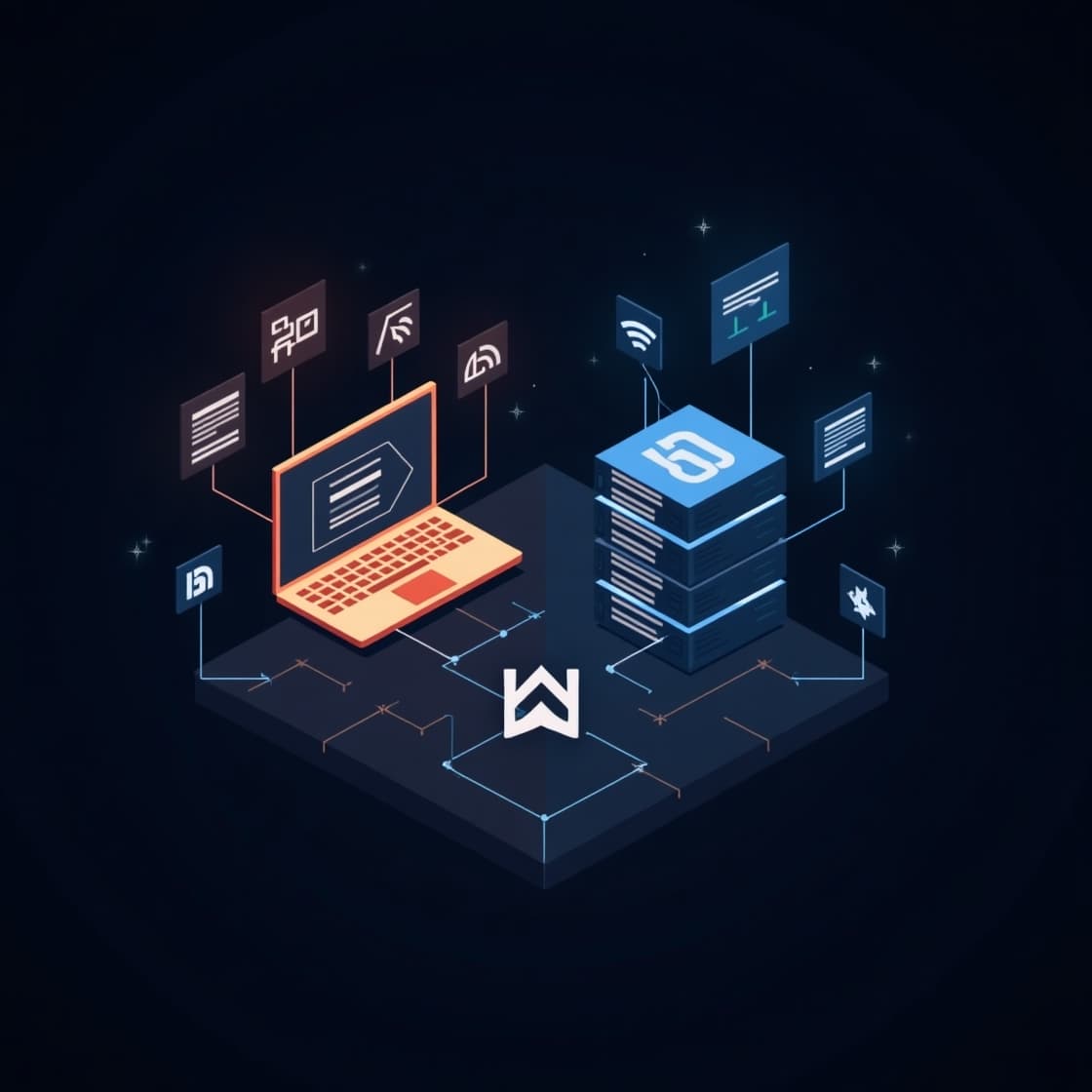
- 30 Views
What is the Difference Between CSR and SSR in Next.js?
Introduction to CSR and SSR in Next.js
When building web applications with Next.js, one of the key decisions you need to make is whether to render your content on the client side (CSR) or the server side (SSR). Both approaches come with their own set of advantages and challenges, and the choice between them can significantly impact the performance and user experience of your application. In this post, we'll explain the differences between CSR and SSR in Next.js and when to use each approach.
Key Concepts to Understand:
- Client-Side Rendering (CSR) - Rendering the web page on the browser.
- Server-Side Rendering (SSR) - Rendering the web page on the server before it reaches the browser.
What is Client-Side Rendering (CSR)?
Client-Side Rendering (CSR) is a technique where the browser (client) fetches the necessary HTML, CSS, and JavaScript and renders the page. This approach is commonly used in Single Page Applications (SPAs), where the page does not require full-page reloads. CSR is typically handled by JavaScript frameworks like React, Angular, and Vue.js.
How CSR Works in Next.js:
- Next.js uses `useEffect` or hooks like `useState` to trigger client-side rendering.
- JavaScript is executed in the browser, and dynamic content is rendered after the initial page load.
- The first page load may be slower because the browser needs to download the JavaScript bundle before rendering the content.
What is Server-Side Rendering (SSR)?
Server-Side Rendering (SSR) is when the HTML is generated on the server and sent to the client, instead of relying on the browser to build the page dynamically. With SSR, the browser receives fully-rendered HTML content, which can help improve the initial load time and the SEO of your web application.
How SSR Works in Next.js:
- Next.js provides functions like `getServerSideProps()` to render pages on the server before sending them to the client.
- On each request, the server generates the HTML, making the content immediately available to the browser.
- SSR helps in scenarios where SEO or fast initial load times are critical, as the content is rendered before it's sent to the browser.
Key Differences Between CSR and SSR in Next.js
While both CSR and SSR can be used to render web pages, they each come with distinct differences. Understanding when to use each approach is essential for building an optimal web app. Below are some key differences between CSR and SSR in Next.js.
CSR vs SSR: Key Differences
- CSR renders the content in the browser, while SSR renders the content on the server.
- CSR can lead to slower initial page loads since the browser has to download and execute JavaScript before rendering, while SSR provides a fully rendered page on the first load.
- SEO: SSR has better SEO performance because search engines can crawl the server-rendered HTML, while CSR relies on client-side rendering, which may cause issues for search engines.
- User experience: CSR allows for a faster subsequent page navigation experience, as only the required data is loaded dynamically without reloading the entire page.
- SSR provides better performance for the first page load, as content is pre-rendered on the server and sent to the client.
When to Use CSR in Next.js?
Client-Side Rendering is best suited for applications where fast interactivity, user engagement, and navigation are prioritized. If your application has dynamic content that frequently changes, CSR ensures that only necessary content is loaded, making it ideal for Single Page Applications (SPAs).
Scenarios Where CSR is Beneficial:
- Dynamic content that doesn't require SEO optimization.
- Applications with frequent user interactions, like dashboards or social media platforms.
- Projects where you need a highly interactive user interface without waiting for the server to render the content.
When to Use SSR in Next.js?
Server-Side Rendering is ideal for applications that require fast initial page loads, better SEO, or content that changes frequently but needs to be indexed by search engines. SSR provides a fully rendered page to the client, making it beneficial for marketing websites, blogs, e-commerce platforms, and other SEO-sensitive applications.
Scenarios Where SSR is Beneficial:
- Websites that need high SEO performance, such as blogs, news sites, or marketing sites.
- Websites where the content changes frequently, and you need to ensure that the latest version is displayed to users.
- E-commerce platforms where products, reviews, and prices are indexed for search engines.
Combining CSR and SSR with Next.js
Next.js allows developers to combine CSR and SSR to get the benefits of both. For example, you can use SSR for the first page load to get a fully rendered page for SEO, and then rely on CSR for subsequent interactions to speed up the user experience. This approach is called hybrid rendering.
How to Use Hybrid Rendering in Next.js:
- Use SSR for critical pages like the homepage, product pages, or blogs.
- Use CSR for user-specific data, like dashboards or profiles that require frequent updates.
Conclusion
Understanding the difference between CSR and SSR is crucial when developing applications in Next.js. While SSR provides faster initial load times and better SEO, CSR offers a more dynamic, interactive experience. By combining both approaches, you can optimize the performance, SEO, and user experience of your Next.js application.